Introduction
401 Unauthorized errors are a common problem encountered when working with REST APIs, especially when dealing with third-party services or your own application’s authentication system. These errors indicate that the request sent to the server lacks valid credentials or the credentials are invalid. Whether you are developing a web app or integrating with an external API, dealing with 401 Unauthorized API errors is part of the process. We will cover both the handling and prevention of 401 Unauthorized API errors in detail. We will discuss common causes, best practices for secure login flows, and proactive measures to ensure smooth user authentication. Let’s dive in.
Understanding 401 Unauthorized Errors
A 401 Unauthorized error occurs when the client fails to provide the necessary authentication or the server cannot validate the provided credentials. This error is related to the authentication process and is typically caused by either missing or incorrect login information.
In the case of APIs, a 401 Unauthorized error could happen for various reasons:
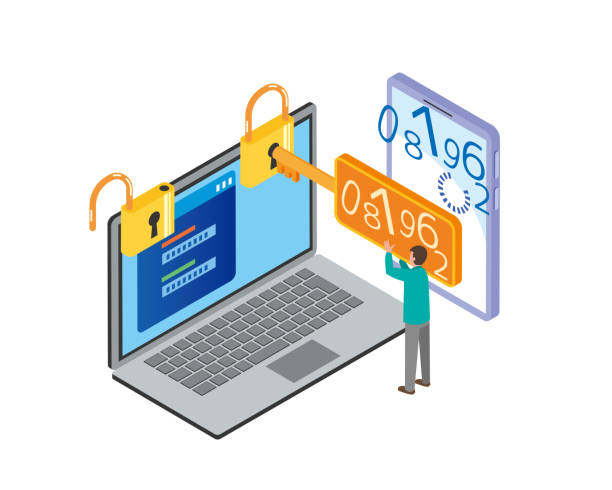
- Invalid API keys or tokens.
- Expired tokens or sessions.
- Missing or malformed Authorization headers in the request.
For developers, handling these errors efficiently ensures that users are guided toward resolving the issue quickly, leading to a smoother user experience.
Handling 401 Unauthorized API Errors
Properly Handle Authentication Failures
The first step in handling 401 Unauthorized errors is to catch the error and display a user-friendly message. When an API request fails due to authentication, it is essential to provide users with clear instructions on how to resolve the issue. Instead of showing a generic error message, inform the user that the issue is related to their authentication credentials.
For example, a simple message such as:
“Your session has expired. Please log in again to continue.”
This helps users understand the cause of the problem and directs them to take action (re-authenticate).
Re-authenticate the User
Once a 401 Unauthorized error is triggered, the most common solution is to re-authenticate the user. This can involve logging in again, refreshing tokens, or obtaining a new set of credentials. The key here is to make this process as seamless as possible.
For web applications, it’s best practice to automatically redirect the user to the login page if their session expires. For API-based applications, you can implement a token refresh mechanism that issues a new token when the old one expires.
How to re-authenticate:
- Revalidate Credentials: Ask users to input their username and password again, or allow them to authenticate using an alternative method (e.g., Google login or OAuth).
- Token Refresh: Use OAuth or JWT tokens to refresh expired tokens without requiring the user to manually log in again.
Log and Monitor Authentication Issues
For developers, keeping track of 401 Unauthorized errors is crucial for identifying patterns and troubleshooting issues. Ensure that the server logs these errors with enough detail to help you debug. Logs should include information such as:
- Timestamp of the error.
- IP address of the user making the request.
- Authentication method used.
- Error code and relevant details.
Test Authentication Requests
Before deploying your application, it’s essential to test authentication mechanisms rigorously. This helps ensure that the application correctly identifies 401 Unauthorized errors and handles them appropriately.
Testing should include scenarios like:
- Validating correct and incorrect API keys.
- Testing expired tokens and token refresh mechanisms.
- Verifying that the correct headers are passed in API requests.
Testing also ensures that users get clear and actionable messages when they face authentication errors.
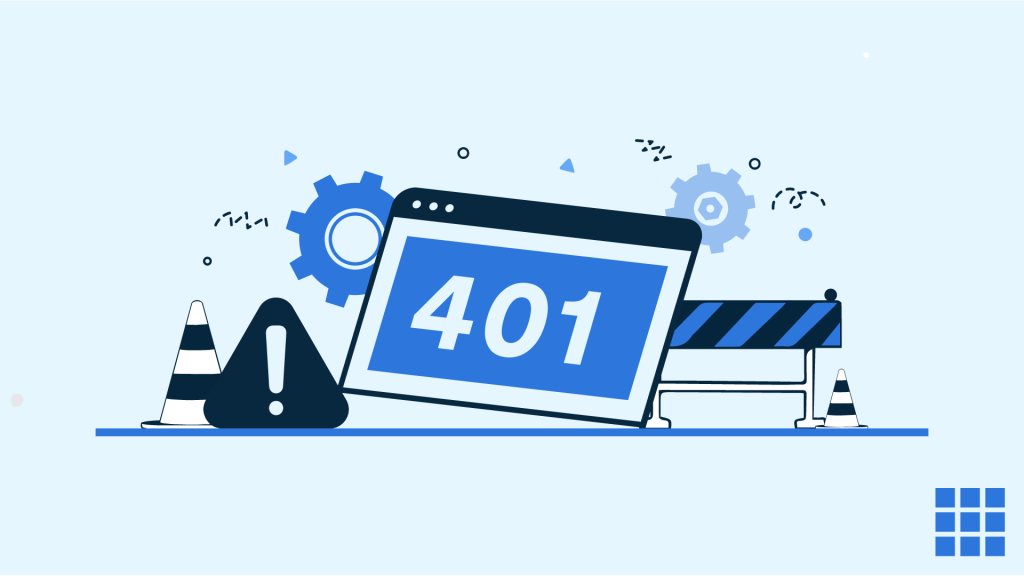
How to Prevent 401 Unauthorized Errors on Your Website
Focus on Secure Login Flows
One of the best ways to prevent 401 Unauthorized errors is to implement a secure login flow. This minimizes the chances of credential-related errors and ensures that only authorized users can access sensitive data.
Some strategies for a secure login flow include:
- Strong Password Policies: Enforce complex password requirements to prevent easily guessable passwords.
- Two-Factor Authentication (2FA): Adding an extra layer of security helps verify the identity of users.
- Password Recovery Mechanism: Allow users to reset their passwords in case they forget their credentials.
- Session Timeouts: Automatically log users out after a certain period of inactivity to reduce security risks.
Manage User Sessions Effectively
User session management is a key part of preventing 401 Unauthorized errors. Sessions should be stored securely, and session tokens must be protected to ensure they are not hijacked. Here are some best practices:
- Use Secure Cookies: Store session tokens in secure, HttpOnly cookies to prevent them from being accessed via JavaScript.
- Session Expiry: Implement session expiration after a set amount of time to limit the risks associated with stale tokens.
- Token Revocation: Provide a mechanism for users to manually log out, which invalidates the session token.
- SameSite Cookies: Use the SameSite cookie attribute to prevent cross-site request forgery (CSRF) attacks.
Implement Proper Authentication Headers
Properly formatting and managing authentication headers in your API requests is crucial for preventing 401 Unauthorized errors. The Authorization header should be correctly constructed and included in every API request that requires authentication.
For instance, if you’re using Bearer tokens for authentication, the header should look like this:
makefile
CopyEdit
Authorization: Bearer <your_token_here>
Ensure that the token is valid and not expired before sending the request. This can be automated with middleware in your application to handle token validation.
Regularly Refresh API Tokens
In APIs that rely on token-based authentication, tokens should have expiration times. When these tokens expire, a 401 Unauthorized error will be returned. To avoid this, implement token refresh mechanisms, which allow the user to refresh their token without logging in again.
If using OAuth authentication, make sure the refresh token is valid and that your system can automatically renew access tokens as needed.
Key Takeaways
Why Handling 401 Errors Properly Matters
A 401 Unauthorized error signals that a user’s credentials are either missing, incorrect, or expired. How you handle this error can significantly impact the user experience. Promptly identifying the error and presenting users with clear, actionable steps can ensure minimal disruption to their experience.
By focusing on secure login flows, session management, and proper token handling, you can prevent 401 Unauthorized errors from occurring in the first place. Additionally, implementing detailed error handling and logging will ensure that you can quickly diagnose and fix issues when they arise.
- 401 Unauthorized Errors occur when the server cannot authenticate the user. This could be due to incorrect credentials, expired tokens, or missing authentication headers.
- Handling 401 errors involves re-authenticating the user, providing clear error messages, logging authentication issues, and testing the authentication flow thoroughly.
- Preventing 401 Unauthorized Errors requires implementing secure login flows, managing sessions properly, ensuring proper authentication headers, and refreshing tokens when necessary.
- Proactive measures like strong password policies, session timeouts, and two-factor authentication can go a long way in preventing these errors.